How To Find The Longest Word In A String
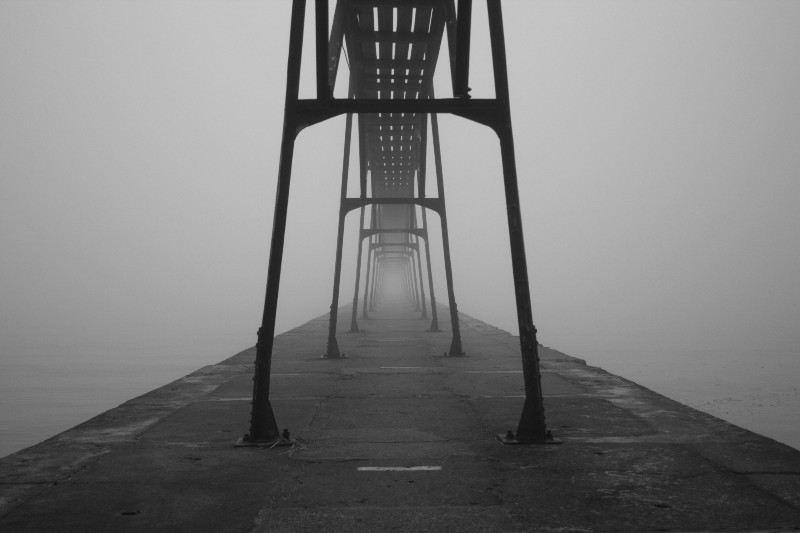
This article is based on Free Lawmaking Camp Basic Algorithm Scripting "Find the Longest Discussion in a String".
In this algorithm, we want to look at each individual word and count how many letters are in each. Then, compare the counts to determine which word has the nearly characters and render the length of the longest give-and-take.
In this article, I'm going to explicate three approaches. Beginning with a FOR loop, 2d using the sort() method, and third using the reduce() method.
Algorithm Claiming
Return the length of the longest word in the provided sentence.Your response should be a number.
Provided examination cases
- findLongestWord("The quick chocolate-brown fox jumped over the lazy canis familiaris") should render a number
- findLongestWord("The quick brownish pull a fast one on jumped over the lazy domestic dog") should return half-dozen
- findLongestWord("May the forcefulness be with you") should return 5
- findLongestWord("Google practise a barrel roll") should render 6
- findLongestWord("What is the average airspeed velocity of an unladen consume") should return eight
- findLongestWord("What if we endeavor a super-long word such every bit otorhinolaryngology") should return 19
function findLongestWord(str) { return str.length; } findLongestWord("The quick brown fox jumped over the lazy dog");
1. Find the Longest Word With a FOR Loop
For this solution, we will use the String.prototype.split() method
- The separate() method splits a Cord object into an assortment of strings by separating the cord into sub strings.
Nosotros will need to add an empty space betwixt the parenthesis of the dissever() method,
var strSplit = "The quick dark-brown play a joke on jumped over the lazy dog".split(' ');
which will output an array of separated words:
var strSplit = ["The", "quick", "brown", "trick", "jumped", "over", "the", "lazy", "domestic dog"];
If yous don't add the infinite in the parenthesis, you lot will have this output:
var strSplit = ["T", "h", "due east", " ", "q", "u", "i", "c", "g", " ", "b", "r", "o", "w", "north", " ", "f", "o", "x", " ", "j", "u", "m", "p", "e", "d", " ", "o", "v", "e", "r", " ", "t", "h", "e", " ", "50", "a", "z", "y", " ", "d", "o", "chiliad"];
part findLongestWord(str) { // Footstep ane. Divide the string into an array of strings var strSplit = str.split(' '); // var strSplit = "The quick brown fox jumped over the lazy dog".split(' '); // var strSplit = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog"]; // Stride ii. Initiate a variable that will concord the length of the longest word var longestWord = 0; // Footstep 3. Create the FOR loop for(var i = 0; i < strSplit.length; i++){ if(strSplit[i].length > longestWord){ // If strSplit[i].length is greater than the word it is compared with... longestWord = strSplit[i].length; // ...so longestWord takes this new value } } /* Here strSplit.length = ix For each iteration: i = ? i < strSplit.length? i++ if(strSplit[i].length > longestWord)? longestWord = strSplit[i].length 1st iteration: 0 yes i if("The".length > 0)? => if(3 > 0)? longestWord = 3 2nd iteration: 1 yes 2 if("quick".length > 3)? => if(5 > three)? longestWord = five 3rd iteration: ii yeah 3 if("dark-brown".length > 5)? => if(5 > 5)? longestWord = 5 4th iteration: iii yes 4 if("fox".length > five)? => if(3 > 5)? longestWord = five 5th iteration: 4 yes 5 if("jumped".length > 5)? => if(6 > 5)? longestWord = half-dozen 6th iteration: five yes 6 if("over".length > 6)? => if(iv > vi)? longestWord = 6 7th iteration: vi yeah 7 if("the".length > 6)? => if(3 > 6)? longestWord = half-dozen 8th iteration: 7 yes 8 if("lazy".length > 6)? => if(4 > 6)? longestWord = 6 9th iteration: 8 yes ix if("dog".length > vi)? => if(3 > half dozen)? longestWord = half dozen tenth iteration: 9 no End of the FOR Loop*/ //Step four. Return the longest word render longestWord; // 6 } findLongestWord("The quick brown fox jumped over the lazy dog");
function findLongestWord(str) { var strSplit = str.split up(' '); var longestWord = 0; for(var i = 0; i < strSplit.length; i++){ if(strSplit[i].length > longestWord){ longestWord = strSplit[i].length; } } return longestWord; } findLongestWord("The quick brown trick jumped over the lazy canis familiaris");
2. Find the Longest Word With the sort() Method
For this solution, we volition use the Array.prototype.sort() method to sort the array by some ordering criterion and and so return the length of the first element of this array.
- The sort() method sorts the elements of an array in identify and returns the array.
In our instance, if we just sort the array
var sortArray = ["The", "quick", "brown", "fob", "jumped", "over", "the", "lazy", "dog"].sort();
we will have this output:
var sortArray = ["The", "brownish", "domestic dog", "fox", "jumped", "lazy", "over", "quick", "the"];
In Unicode, numbers come up before upper example letters, which come earlier lower instance letters.
We demand to sort the elements by some ordering criterion,
[].sort(role(firstElement, secondElement) { return secondElement.length — firstElement.length; })
where the length of the 2d element is compared to the length of the first element in the array.
role findLongestWord(str) { // Pace 1. Split up the cord into an assortment of strings var strSplit = str.split(' '); // var strSplit = "The quick brown pull a fast one on jumped over the lazy dog".split up(' '); // var strSplit = ["The", "quick", "brownish", "fox", "jumped", "over", "the", "lazy", "domestic dog"]; // Step 2. Sort the elements in the array var longestWord = strSplit.sort(role(a, b) { return b.length - a.length; }); /* Sorting process a b b.length a.length var longestWord "The" "quick" 5 three ["quick", "The"] "quick" "brown" 5 v ["quick", "brownish", "The"] "brown" "fob" three 5 ["quick", "brownish", "The", "pull a fast one on"] "play a joke on" "jumped" six three ["jumped", quick", "dark-brown", "The", "play a trick on"] "jumped" "over" four six ["jumped", quick", "brown", "over", "The", "play a joke on"] "over" "the" iii 4 ["jumped", quick", "brown", "over", "The", "fox", "the"] "the" "lazy" iv 3 ["jumped", quick", "brown", "over", "lazy", "The", "flim-flam", "the"] "lazy" "dog" iii 4 ["jumped", quick", "brown", "over", "lazy", "The", "fox", "the", "domestic dog"] */ // Footstep iii. Return the length of the starting time element of the array return longestWord[0].length; // var longestWord = ["jumped", "quick", "brown", "over", "lazy", "The", "fox", "the", "canis familiaris"]; // longestWord[0]="jumped" => jumped".length => 6 } findLongestWord("The quick brown flim-flam jumped over the lazy canis familiaris");
role findLongestWord(str) { var longestWord = str.separate(' ').sort(part(a, b) { return b.length - a.length; }); return longestWord[0].length; } findLongestWord("The quick chocolate-brown fox jumped over the lazy dog");
iii. Find the Longest Give-and-take With the reduce() Method
For this solution, we will use the Assortment.prototype.reduce().
- The reduce() method applies a function confronting an accumulator and each value of the array (from left-to-right) to reduce information technology to a single value.
reduce() executes a callback function in one case for each element nowadays in the assortment.
You can provide an initial value as the 2nd argument to reduce, here we will add an empty string "".
[].reduce(function(previousValue, currentValue) {...}, "");
function findLongestWord(str) { // Step 1. Dissever the string into an assortment of strings var strSplit = str.carve up(' '); // var strSplit = "The quick brown fox jumped over the lazy dog".split(' '); // var strSplit = ["The", "quick", "brown", "flim-flam", "jumped", "over", "the", "lazy", "dog"]; // Step 2. Use the reduce method var longestWord = strSplit.reduce(role(longest, currentWord) { if(currentWord.length > longest.length) return currentWord; else return longest; }, ""); /* Reduce process currentWord longest currentWord.length longest.length if(currentWord.length > longest.length)? var longestWord "The" "" 3 0 aye "The" "quick" "The" 5 3 aye "quick" "dark-brown" "quick" 5 5 no "quick" "fox" "quick" three 5 no "quick" "jumped" "quick" 6 5 yes "jumped" "over" "jumped" 4 6 no "jumped" "the" "jumped" 3 6 no "jumped" "lazy" "jumped" 4 half dozen no "jumped" "dog" "jumped" three half-dozen no "jumped" */ // Step iii. Return the length of the longestWord return longestWord.length; // var longestWord = "jumped" // longestWord.length => "jumped".length => 6 } findLongestWord("The quick brown pull a fast one on jumped over the lazy canis familiaris");
function findLongestWord(str) { var longestWord = str.split(' ').reduce(office(longest, currentWord) { render currentWord.length > longest.length ? currentWord : longest; }, ""); return longestWord.length; } findLongestWord("The quick dark-brown fob jumped over the lazy dog");
I hope you plant this helpful. This is part of my "How to Solve FCC Algorithms" serial of articles on the Complimentary Lawmaking Campsite Algorithm Challenges, where I propose several solutions and explain step-by-step what happens under the hood.
Iii means to repeat a cord in JavaScript
In this article, I'll explain how to solve freeCodeCamp'due south "Echo a string repeat a string" claiming. This involves…
Two ways to ostend the catastrophe of a String in JavaScript
In this commodity, I'll explain how to solve freeCodeCamp's "Ostend the Ending" challenge.
Three Ways to Reverse a String in JavaScript
This article is based on Free Code Camp Basic Algorithm Scripting "Reverse a Cord"
Three Ways to Factorialize a Number in JavaScript
This commodity is based on Complimentary Lawmaking Camp Basic Algorithm Scripting "Factorialize a Number"
Ii Ways to Check for Palindromes in JavaScript
This article is based on Free Code Camp Basic Algorithm Scripting "Check for Palindromes".
Three Ways to Title Case a Sentence in JavaScript
This article is based on Costless Code Camp Basic Algorithm Scripting "Championship Case a Sentence".
Iii ways you can detect the largest number in an array using JavaScript
In this article, I'grand going to explicate how to solve Free Code Camp's "Render Largest Numbers in Arrays" challenge. This…
If you have your own solution or any suggestions, share them below in the comments.
Or y'all tin can follow me on Medium , Twitter, Github and LinkedIn, correct after yous click the green middle beneath ;-)
#StayCurious, #KeepOnHacking & #MakeItHappen!
Resources
- dissever() method — MDN
- sort() method — MDN
- reduce() — MDN
- Cord.length — MDN
- for — MDN
Acquire to lawmaking for gratis. freeCodeCamp's open source curriculum has helped more than forty,000 people get jobs as developers. Get started
Source: https://www.freecodecamp.org/news/three-ways-to-find-the-longest-word-in-a-string-in-javascript-a2fb04c9757c/
Posted by: tatetheyeary.blogspot.com
0 Response to "How To Find The Longest Word In A String"
Post a Comment